前言
从本篇开始我们就要进入执行引擎的学习
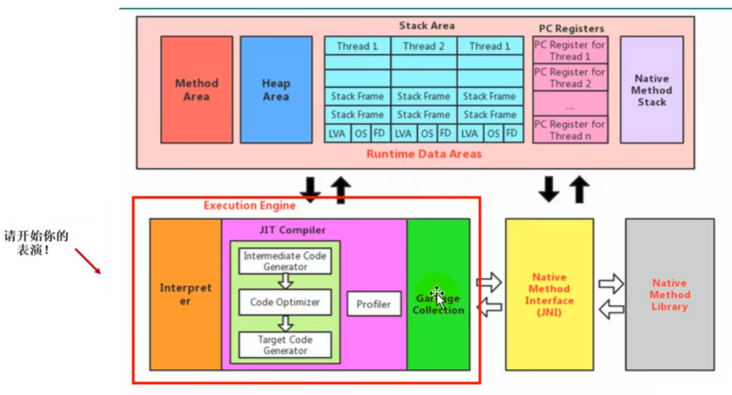
一、执行引擎概述
我们说执行引擎是Java虚拟机核心的组成部分之一
其实“虚拟机”是一个相对于“物理机”的概念,这两种机器都有代码执行能力,其区别是物理机的执行引擎是直接建立在处理器、缓存、指令集和操作系统层面上
的,而虚拟机的执行引擎则是由软件自行实现的,因此可以不受物理条件制约地定制指令集与执行引擎的结构体系,能够执行那些不被硬件直接支持的指令集格式
JVM的主要任务是负责装载字节码到其内部
,但字节码并不能够直接运行在操作系统之上,因为字节码指令并非等价于本地机器指令,它内部包含的仅仅只是一些能够被JVM所识别的字节码指令、符号表,以及其他辅助信息
那么如果想要让一个Java程序运行起来,执行引擎(Execution Engine)的任务就是将字节码指令解释/编译为对应平台上的本地机器指令才可以
。简单来说,JVM中的执行引擎充当了将高级语言翻译为机器语言的译者
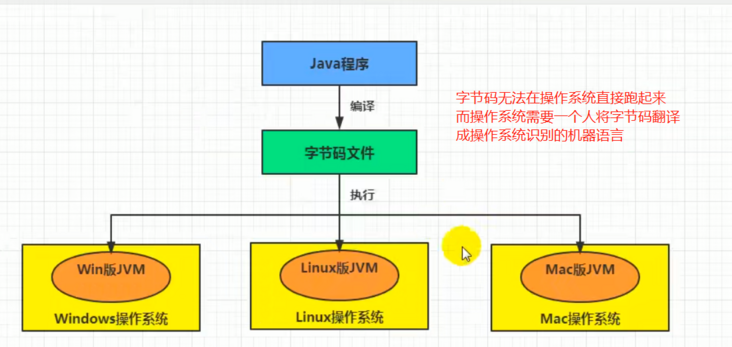
举个例子:美国与韩国进行交流时,美国讲英语,韩国讲韩语,中间就需要一个人进行翻译
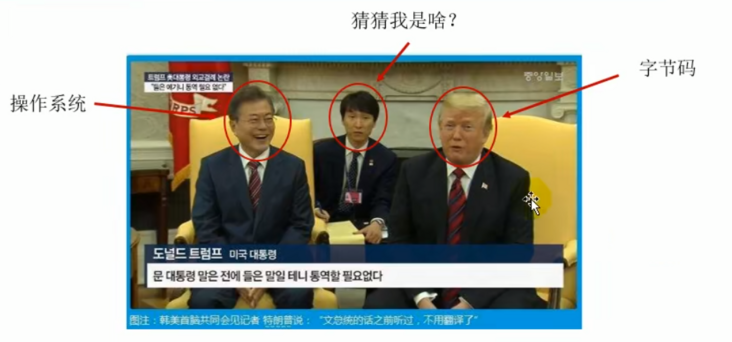
执行引擎的工作过程
================================
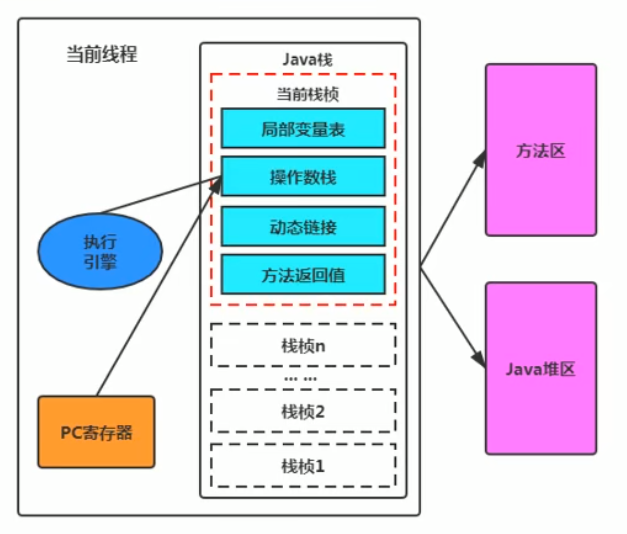
执行引擎在执行的过程中究竟需要执行什么样的字节码指令完全依赖于PC寄存器
每当执行完一项指令操作后,PC寄存器就会更新下一条需要被执行的指令地址
当然方法在执行的过程中,执行引擎有可能会通过存储在局部变量表中的对象引用准确定位到存储在Java堆区中的对象实例信息,以及通过对象头中的元数据指针定位到目标对象的类型信息
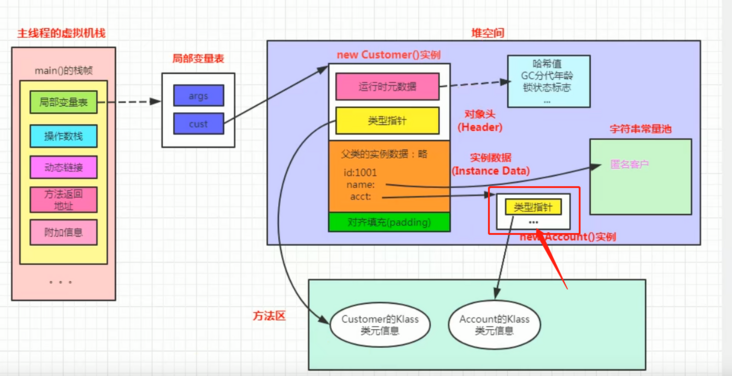
从外观上来看,所有的Java虚拟机的执行引擎输入、处理、输出都是一致的:输入的是字节码二进制流,处理过程是字节码解析执行、即时编译的等效过程,输出的是执行过程
二、Java代码编译和执行过程
大部分的程序代码转换成物理机的目标代码或虚拟机能执行的指令集之前
,都需要经过下图中的各个步骤:
- 橙色部分是
编译生成生成字节码文件的过程
(javac编译器来完成,也就是前端编译器),和JVM没有关系。 - 绿色(解释执行)和蓝色(即时编译)才是JVM需要考虑的过程
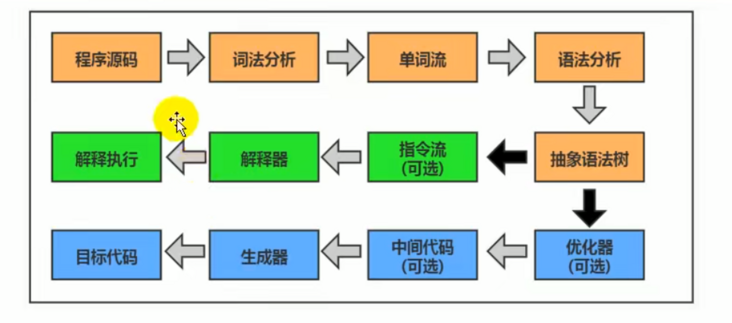
而我们的前端编译器JavaC生成字节码文件的执行过程如下图:
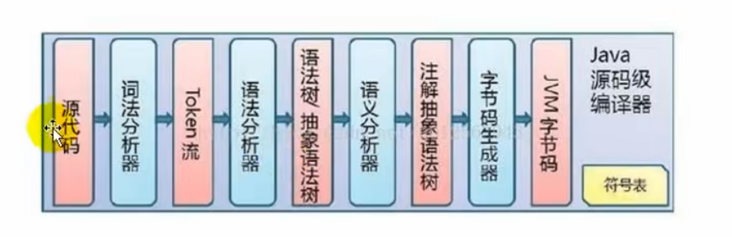
当我们有字节码文件后就交给JVM考虑绿色与蓝色的部分,流程执行图如下:
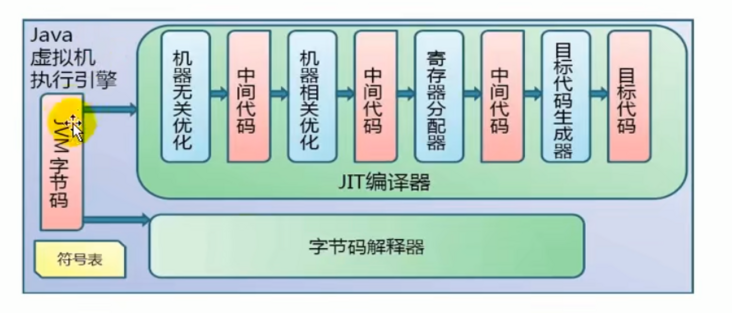
当有了上面两个图后,再遇到什么是解释器?什么是JIT编译器?
这个问题是不是就可以给出答案了
解释器:当Java虚拟机启动时会根据预定义的规范对字节码采用逐行解释的方式执行,将每条字节码文件中的内容“翻译”为对应平台的本地机器指令执行
JIT(Just In Time Compiler)编译器:就是虚拟机将源代码一次性直接编译成和本地机器平台相关的机器语言,但并不是马上执行
那么还有一个问题仅接着出现了:为什么Java是半编译半解释型语言?
原JDK1.0时代,将Java语言定位为“解释执行”还是比较准确的。
后来Java也发展出可以直接生成本地代码的编译器。即JVM在执行Java代码的时候,通常都会将解释执行与编译执行二者结合起来进行
错误理解Java是半编译半解释型语言

半编译半解释语言的主要原因是执行引擎在解释字节码文件的时候,即可以使用解释器又可以使用JIT编译器
JIT编译器将字节码翻译成本地(机器指令)代码后,就可以做一个缓存操作
,存储在方法区的JIT 代码缓存中(执行效率更高了),并且在翻译成本地代码的过程中可以做优化
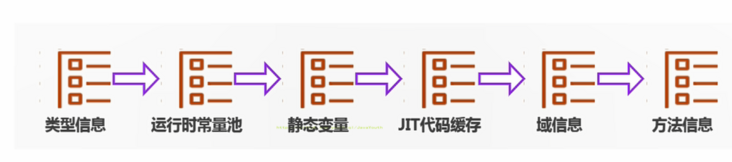
我们总的来说就是可以用下图总结一下
三、机器码、指令、汇编语言
接下来我们介绍一下机器码、指令、汇编语言等等信息
机器码是什么?
================================
各种用二进制编码方式表示的指令,叫做机器指令码。开始人们就用它采编写程序,这就是机器语言
机器语言虽然能够被计算机理解和接受,但和人们的语言差别太大,不易被人们理解和记忆,并且用它编程容易出差错
用它编写的程序一经输入计算机CPU直接读取运行,因此和其他语言编的程序相比,执行速度最快
机器指令与CPU紧密相关,所以不同种类的CPU所对应的机器指令也就不同
指令是什么?
================================
由于机器码是由0和1组成的二进制序列,可读性实在太差,所以就发明了指令
指令就是把机器码中特定的0和1序列,简化成对应的指令(一般为英文简写,如mov,inc等),可读性稍好
由于不同的硬件平台,执行同一个操作,对应的机器码可能不同,所以不同的硬件平台的同一种指令(比如mov),对应的机器码也可能不同
不同的硬件平台,各自支持的指令是有差别的,如常见的
- x86指令集,对应的是x86架构的平台
- ARM指令集,对应的是ARM架构的平台
汇编语言是什么?
================================
由于指令的可读性还是太差,于是人们又发明了汇编语言
在汇编语言中,用助记符(Mnemonics)代替机器指令的操作码,用地址符号(Symbol)或标号(Label)代替指令或操作数的地址
在不同的硬件平台,汇编语言对应着不同的机器语言指令集,通过汇编过程转换成机器指令
由于计算机只认识指令码,所以用汇编语言编写的程序还必须翻译(汇编)成机器指令码,计算机才能识别和执行
高级语言是什么?
================================
为了使计算机用户编程序更容易些,后来就出现了各种高级计算机语言。高级语言比机器语言、汇编语言更接近人的语言
当计算机执行高级语言编写的程序时,仍然需要把程序解释和编译成机器的指令码。完成这个过程的程序就叫做解释程序或编译程序
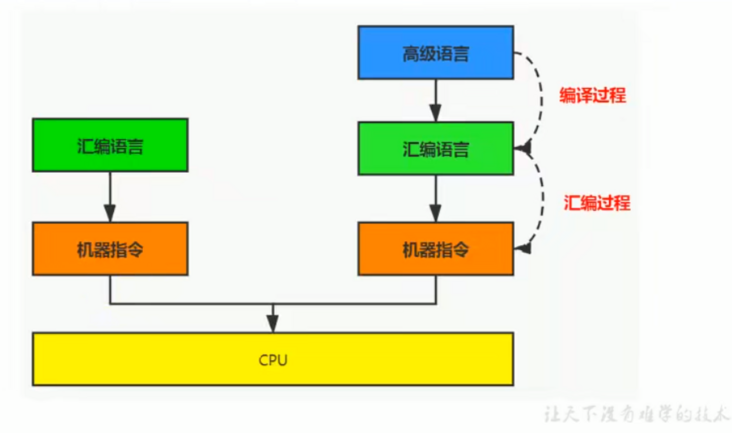
C、C++源程序执行过程
================================
我们上面说到高级语言翻译成汇编称为编译过程,汇编翻译成机器称为汇编过程
所以过程又可以分成两个阶段:编译和汇编
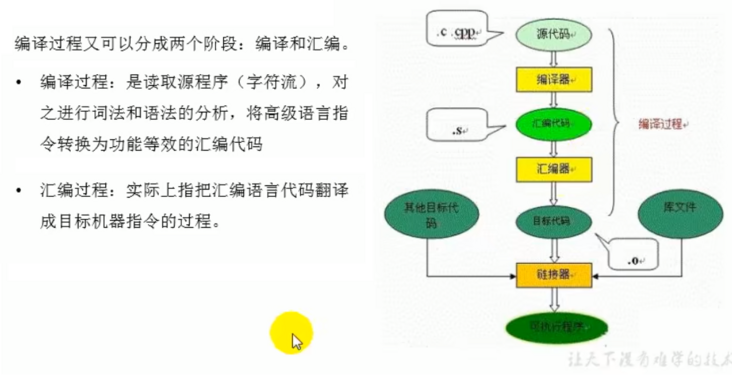
字节码是什么?
================================
字节码是一种中间状态(中间码)的二进制代码(文件),它比机器码更抽象,需要直译器转译后才能成为机器码
字节码主要为了实现特定软件运行和软件环境、与硬件环境无关
字节码的实现方式是通过编译器和虚拟机器。编译器将源码编译成字节码,特定平台上的虚拟机器将字节码转译为可以直接执行的指令
字节码典型的应用为:Java bytecode
比如说我们之前调到的CA FE BA BE
四、解释器
接下来我们就开始介绍解释器,那么为什么要有解释器,其实主要还是
JVM设计者们的初衷仅仅只是单纯地为了满足Java程序实现跨平台特性,因此避免采用静态编译的方式由高级语言直接生成本地机器指令,从而诞生了实现解释器在运行时采用逐行解释字节码执行程序的想法
(也就是产生了一个中间产品字节码)
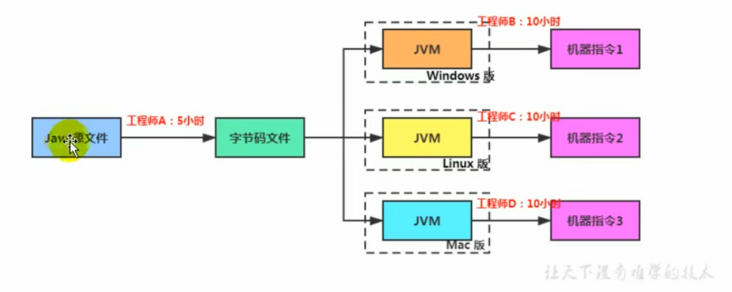
解释器真正意义上所承担的角色就是一个运行时“翻译者”,将字节码文件中的内容“翻译”为对应平台的本地机器指令执行
当一条字节码指令被解释执行完成后,接着再根据PC寄存器中记录的下一条需要被执行的字节码指令执行解释操作
解释器分类
================================
在Java的发展历史里,一共有两套解释执行器即
- 古老的字节码解释器
- 现在普遍使用的模板解释器
字节码解释器在执行时通过纯软件代码模拟字节码的执行,效率非常低下
而模板解释器将每一条字节码和一个模板函数相关联,模板函数中直接产生这条字节码执行时的机器码,从而很大程度上提高了解释器的性能
在HotSpot VM中,解释器主要由Interpreter模块和Code模块构成
- Interpreter模块:实现了解释器的核心功能
- Code模块:用于管理HotSpot VM在运行时生成的本地机器指令
解释器的现状
================================
由于解释器在设计和实现上非常简单,因此除了Java语言之外,还有许多高级语言同样也是基于解释器执行的,比如Python、Perl、Ruby等。但是在今天,基于解释器执行已经沦落为低效的代名词,并且时常被一些C/C++程序员所调侃
为了解决这个问题,JVM平台支持一种叫作即时编译的技术。即时编译的目的是避免函数被解释执行,而是将整个函数体编译成为机器码,每次函数执行时,只执行编译后的机器码即可
,这种方式可以使执行效率大幅度提升
不过无论如何基于解释器的执行模式仍然为中间语言
的发展做出了不可磨灭的贡献
五、JIT编译器
前面提到过一个图说橙色属于Javac编译字节码过程,绿色属于解释器过程,蓝色属于JIT编译器过程
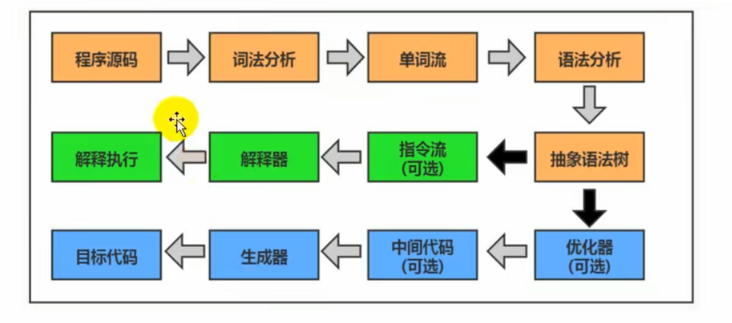
并且也进行了图的总结,如下:
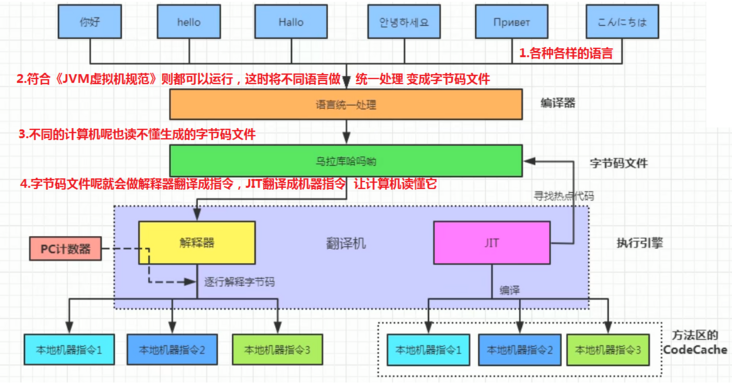
想让其他变成语言运行在Java虚拟机上,我们说会统一做处理编译成字节码文件
并使得本地操作系统与硬件资源运行起来,有两种方式:
- 解释器逐条解释字节码
- 即时编译将字节码文件翻译成机器指令并且缓存起来
接下来我们要讲的就是JIT编译器,我们刚刚对java代码执行做了分类
第一种是将源代码编译成字节码文件,然后在运行时通过解释器将字节码文件转为机器码执行
第二种是编译执行(直接编译成机器码)。现代虚拟机为了提高执行效率,会使用即时编译技术(JIT,Just In Time)将方法编译成机器码后再执行
HotSpot VM是目前市面上高性能虚拟机的代表作之一。它采用解释器与即时编译器并存的架构。在Java虚拟机运行时,解释器和即时编译器能够相互协作,各自取长补短,尽力去选择最合适的方式来权衡编译本地代码的时间和直接解释执行代码的时间
在今天Java程序的运行性能早已脱胎换骨,已经达到了可以和C/C++ 程序一较高下的地步
为啥还需要解释器呢?
================================
有些开发人员会感觉到诧异,既然HotSpot VM中已经内置JIT编译器了,那么为什么还需要再使用解释器来“拖累”程序的执行性能呢?
比如JRockit VM内部就不包含解释器,字节码全部都依靠即时编译器编译后执行
JRockit虚拟机是砍掉了解释器也就是只采及时编译器。
那是因为呢JRockit只部署在服务器上,一般已经有时间让他进行指令编译的过程了,对于响应来说要求不高,等及时编译器的编译完成后,就会提供更好的性能
这时我们就明确下来了
- 当程序启动后,解释器可以马上发挥作用,响应速度快,省去编译的时间,立即执行
- 编译器要想发挥作用,把代码编译成本地代码,需要一定的执行时间,但编译为本地代码后,执行效率高
尽管JRockit VM中程序的执行性能会非常高效,但程序在启动时必然需要花费更长的时间来进行编译。
对于服务端应用来说,启动时间并非是关注重点,但对于那些看中启动时间的应用场景而言,或许就需要采用解释器与即时编译器并存的架构来换取一个平衡点
HotSpot JVM的执行方式
================================
当Java虚拟器启动时,解释器可以首先发挥作用
,而不必等待即时编译器全部编译完成后再执行,这样可以省去许多不必要的编译时间
。并且随着程序运行时间的推移,即时编译器发挥作用,根据热点探测功能,将有价值的字节码编译成本地机器指令以换取更高的程序执行效率
同时解释执行在编译器进行激进优化不成立的时候,作为编译器的“逃生门”(后备方案)。
注意解释执行与编译执行
在线上环境微妙的辩证关系。机器在热机状态(已经运行了一段时间叫热机状态)可以承受的负载要大于冷机状态(刚启动的时候叫冷机状态)
。如果以热机状态时的流量进行切流,可能使处于冷机状态的服务器因无法承载流量而假死
阿里团队案例
================================
在生产环境发布过程中,根据机器数量划分成多个批次,每个批次的机器数至多占到整个集群的1/8以分批的方式进行发布。
曾经有这样的故障案例:某程序员在发布平台进行分批发布,在输入发布总批数时,误填写成分为两批发布。如果是热机状态,在正常情况下一半的机器可以勉强承载流量,但由于刚启动的JVM均是解释执行,还没有进行热点代码统计和JIT动态编译,导致机器启动之后,当前1/2发布成功的服务器马上全部宕机,此故障说明了JIT的存在
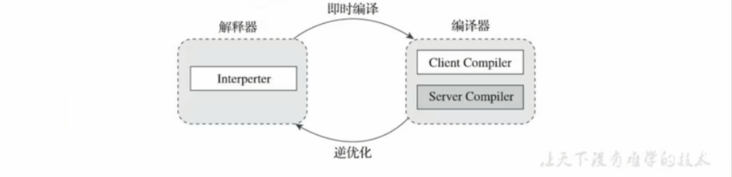
接下来我们使用一个案例,来查看JIT即时编译的次数
public class JITTest { public static void main(String[] args) { ArrayListlist = new ArrayList<>(); for (int i = 0; i < 1000; i++) { list.add("让天下没有难学的技术"); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } }}
当我们运行起来后,可以打开Java VisualVM工具查看该类
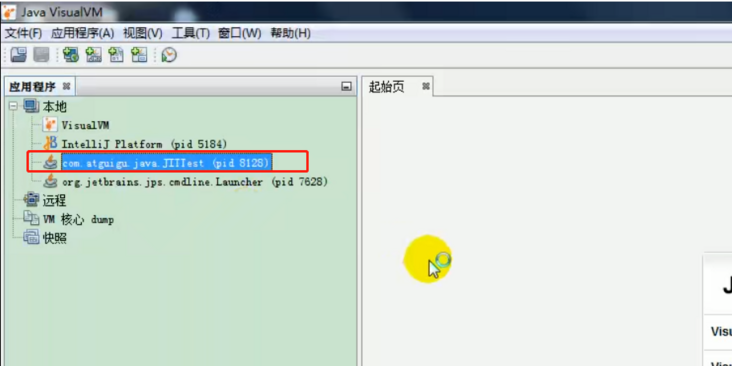
JIT编译器相关概念
================================
Java 语言的“编译期”其实是一段“不确定”的操作过程,因为它
- 可能是指一个前端编译器(其实叫“编译器的前端”更准确一些)把.java文件转变成.class文件的过程
- 可能是指虚拟机的后端运行期编译器(JIT编译器,Just In Time Compiler)把字节码转变成机器码的过程。
典型的编译器:
- 前端编译器:Sun的javac、Eclipse JDT中的增量式编译器(ECJ)。
- JIT编译器:HotSpot VM的C1、C2编译器。
- AOT 编译器:GNU Compiler for the Java(GCJ)、Excelsior JET。
当然也可能是指使用静态提前编译器(AOT编译器,Ahead of Time Compiler)直接把.java文件编译成本地机器代码的过程。(可能是后续发展的趋势)
热点代码及探测方式
================================
当然是否需要启动JIT编译器
将字节码直接编译为对应平台的本地机器指令,则需要根据代码被调用执行的频率而定
关于那些需要被编译为本地代码的字节码,也被称之为“热点代码”
,JIT编译器在运行时会针对那些频繁被调用的“热点代码”做出深度优化,将其直接编译为对应平台的本地机器指令,以此提升Java程序的执行性能
一个被多次调用的方法,或者是一个方法体内部循环次数较多的循环体都可以被称之为“热点代码”
,因此都可以通过JIT编译器编译为本地机器指令。由于这种编译方式发生在方法的执行过程中,因此也被称之为栈上替换,或简称为OSR (On StackReplacement)编译
一个方法究竟要被调用多少次,或者一个循环体究竟需要执行多少次循环
才可以达到这个标准?必然需要一个明确的阈值
,JIT编译器才会将这些“热点代码”编译为本地机器指令执行。
目前HotSpot VM所采用的热点探测方式是基于计数器的热点探测
采用基于计数器的热点探测,HotSpot VM将会为每一个方法都建立2个不同类型的计数器
- 方法调用计数器用于统计方法的调用次数
- 回边计数器则用于统计循环体执行的循环次数
方法调用计数器
这个计数器就用于统计方法被调用的次数,它的默认阀值在Client模式下是1500次,在Server模式下是10000次。超过这个阈值,就会触发JIT编译。
这个阀值可以通过虚拟机参数 -XX:CompileThreshold 来人为设定
当一个方法被调用时,会先检查该方法是否存在被JIT编译过的版本
- 如果存在,则优先使用编译后的本地代码来执行
- 如果不存在已被编译过的版本,则将此方法的调用计数器值加1,然后判断方法调用计数器与回边计数器值之和是否超过方法调用计数器的阀值
如果未超过阈值,则使用解释器对字节码文件解释执行。若如果已超过阈值,那么将会向即时编译器提交一个该方法的代码编译请求。
如果不做任何设置,方法调用计数器统计的并不是方法被调用的绝对次数
,而是一个相对的执行频率,即一段时间之内方法被调用的次数
如果方法的调用次数仍然不足以让它提交给即时编译器编译,那这个方法的调用计数器就会被减少一半,这个过程称为方法调用计数器热度的衰减(Counter Decay),而这段时间就称为此方法统计的半衰周期(Counter Half Life Time)
进行热度衰减的动作是在虚拟机进行垃圾收集时顺便进行的,可以使用虚拟机参数 -XX:-UseCounterDecay 来关闭热度衰减
,让方法计数器统计方法调用的绝对次数
,这样的话只要系统运行时间足够长,绝大部分方法都会被编译成本地代码
使用-XX:CounterHalfLifeTime参数设置半衰周期的时间
,单位是秒
回边计数器
它的作用是统计一个方法中循环体代码执行的次数,
在字节码中遇到控制流向后跳转的指令称为“回边”(Back Edge)。显然建立回边计数器统计的目的就是为了触发OSR编译
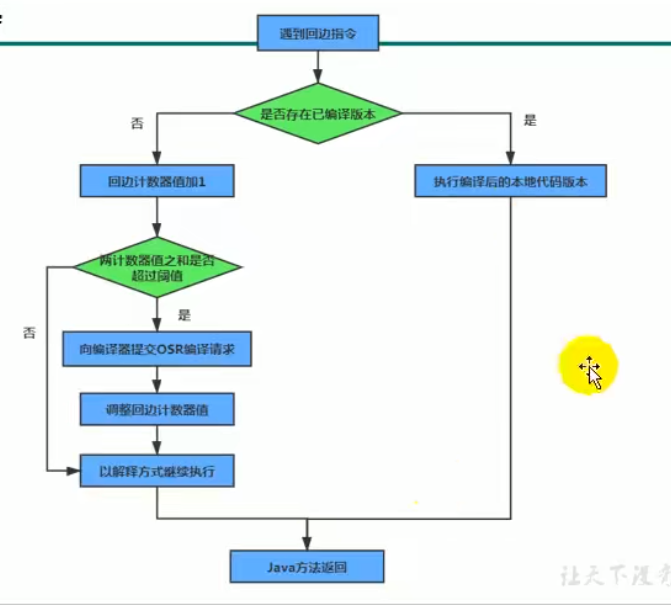
六、HotSpotVm 设置程序执行方式
我们刚刚也说HotSpot VM是采用解释器与即时编译器并存的架构
,当然开发人员可以根据具体的应用场景
,通过命令显式地为Java虚拟机指定在运行时到底是完全采用解释器执行,还是完全采用即时编译器执行
- -Xint:完全采用解释器模式执行程序;
- -Xcomp:完全采用即时编译器模式执行程序。如果即时编译出现问题,解释器会介入执行
- -Xmixed:采用解释器+即时编译器的混合模式共同执行程序
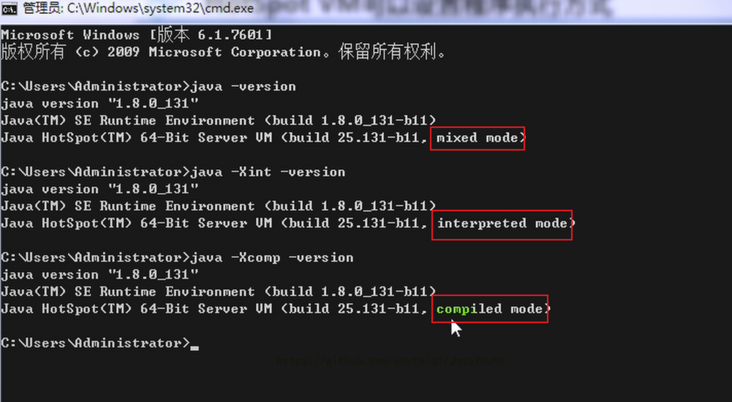
我们也可以使用示例具体体会一下不同模式下的差距是怎么样的
public class IntCompTest { public static void main(String[] args) { long start = System.currentTimeMillis(); testPrimeNumber(1000000); long end = System.currentTimeMillis(); System.out.println("花费的时间为:" + (end - start)); } public static void testPrimeNumber(int count){ for (int i = 0; i < count; i++) { //计算100以内的质数 label:for(int j = 2;j <= 100;j++){ for(int k = 2;k <= Math.sqrt(j);k++){ if(j % k == 0){ continue label; } } //System.out.println(j); } } }}
-Xint:完全采用解释器模式执行程序:
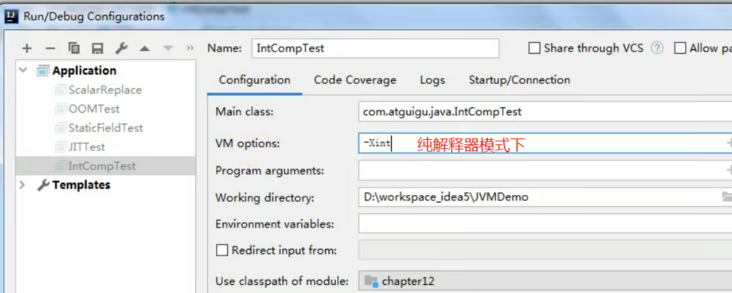
花费的时间为:6520
-Xcomp:完全采用即时编译器模式执行程序:
花费的时间为:950
-Xmixed:采用解释器+即时编译器:
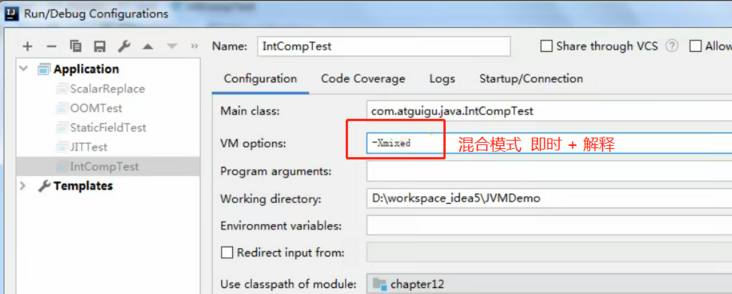
花费的时间为:936
HotSpotVM JIT 分类
================================
在HotSpot VM中内嵌有两个JIT编译器,分别为Client Compiler和Server Compiler,但大多数情况下我们简称为C1编译器 和 C2编译器
开发人员可以通过如下命令显式指定Java虚拟机在运行时到底使用哪一种即时编译器,如下所示:
- -client:指定Java虚拟机运行在Client模式下,并使用C1编译器;
- -server:指定Java虚拟机运行在server模式下,并使用C2编译器
C1编译器会对字节码进行简单和可靠的优化耗时短
,以达到更快的编译速度。C2进行耗时较长的优化,以及激进优化
,但优化的代码执行效率更高。(使用C++)
C1和C2编译器不同的优化策略
================================
在不同的编译器上有不同的优化策略,C1编译器上主要有方法内联,去虚拟化、元余消除。
- 方法内联:将引用的函数代码编译到引用点处这样可以减少栈帧的生成,减少参数传递以及跳转过程
- 去虚拟化:对唯一的实现樊进行内联
- 冗余消除:在运行期间把一些不会执行的代码折叠掉
C2的优化主要是在全局层面,逃逸分析是优化的基础。基于逃逸分析在C2上有如下几种优化:
- 标量替换:用标量值代替聚合对象的属性值
- 栈上分配:对于未逃逸的对象分配对象在栈而不是堆
- 同步消除:清除同步操作,通常指synchronized
一般来说JIT编译出来的机器码性能比解释器高,C2编译器启动长比C1编译器慢,系统稳定执行以后C2编译器远远快于C1编译器
关于AOT 编译器
================================
jdk9引入了AoT编译器(静态提前编译器,Ahead of Time Compiler)
Java 9引入了实验性AOT编译工具jaotc。它借助了Graal编译器,将所输入的Java类文件转换为机器码,并存放至生成的动态共享库之中
所谓AOT编译,是与即时编译相对立的一个概念。我们知道,即时编译指的是在程序的运行过程中,将字节码转换为可在硬件上直接运行的机器码
,并部署至托管环境中的过程。而AOT编译指的则是在程序运行之前,便将字节码转换为机器码的过程
AOT编译器编译器的优缺点
好处:
- Java虚拟机加载已经预编译成二进制库,可以直接执行。
- 不必等待即时编译器的预热,减少Java应用给人带来“第一次运行慢” 的不良体验
缺点:
- 破坏了 java “ 一次编译,到处运行”,必须为每个不同的硬件,OS编译对应的发行包
- 降低了Java链接过程的动态性,加载的代码在编译器就必须全部已知。
还需要继续优化中,最初只支持Linux X64 java base
关于Graal 编译器
================================
自JDK 10起,HotSpot又加入一个全新的即时编译器:Gralal编译器。
编译效果短短几年时间就追评了C2编译器.未来可期.
目前带着“实验状态"标签,需要使用开关参数-XX: +UnlockExperimental VMOptions -XX: +UseJVMCICompiler
去激活,才可以使用。